PHP - Interview Series - 3
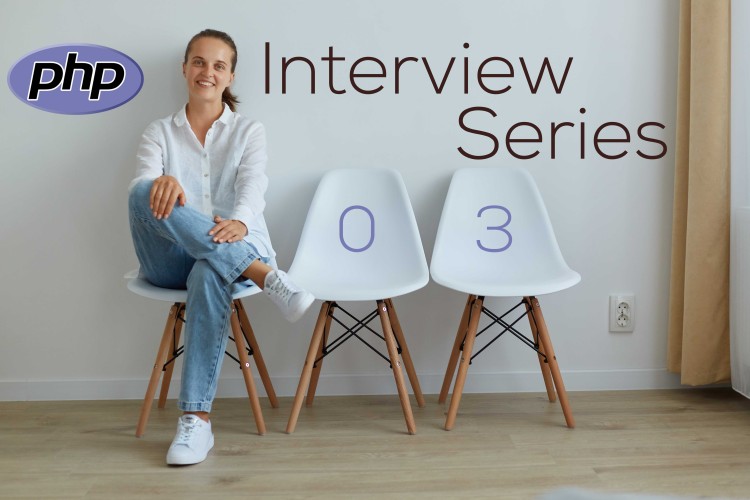
- What is the use of the array_keys() function in PHP?
Answer: The array_keys() function is used to return all the keys or a subset of the keys of an array.
Example code:
$data = array("name" => "John", "age" => 30); $keys = array_keys($data);
- What is the use of the array_values() function in PHP?
Answer: The array_values() function is used to return all the values of an array, without the keys.
Example code:
$data = array("name" => "John", "age" => 30); $values = array_values($data);
- What is the use of the array_unique() function in PHP?
Answer: The array_unique() function is used to remove duplicate values from an array.
Example code:
$numbers = array(1, 2, 3, 2, 4, 5); $unique_numbers = array_unique($numbers);
- What is the use of the array_search() function in PHP?
Answer: The array_search() function is used to search an array for a specific value, and return its corresponding key.
Example code:
$numbers = array(1, 2, 3, 4, 5); $key = array_search(3, $numbers);
- What is the use of the array_flip() function in PHP?
Answer: The array_flip() function is used to exchange the keys and values of an array.
Example code:
$data = array("name" => "John", "age" => 30); $flipped_data = array_flip($data);
- What is the use of the array_rand() function in PHP?
Answer: The array_rand() function is used to randomly select one or more keys from an array.
Example code:
$numbers = array(1, 2, 3, 4, 5); $random_key = array_rand($numbers);
- What is the use of the array_fill() function in PHP?
Answer: The array_fill() function is used to fill an array with a specified value, and a specified number of elements.
Example code:
$filled_array = array_fill(0, 5, "Hello");
- What is the use of the array_chunk() function in PHP?
Answer: The array_chunk() function is used to split an array into chunks of a specified size.
Example code:
$numbers = array(1, 2, 3, 4, 5); $chunks = array_chunk($numbers, 2);
- What is the use of the explode() function in PHP?
Answer: The explode() function is used to split a string into an array, using a specified delimiter.
Example code:
$string = "Hello, World!"; $words = explode(",", $string);
- What is the use of the implode() function in PHP?
Answer: The implode() function is used to join the elements of an array into a string, using a specified delimiter.
Example code:
$words = array("Hello", "World!"); $string = implode(" ", $words);
- What is the use of the preg_match() function in PHP?
Answer: The preg_match() function is used to perform a regular expression match on a string.
Example code:
$string = "The quick brown fox"; $matches = array(); preg_match("/quick/", $string, $matches);