Laravel 10 - A Deep Dive Into the Latest Updates and Features
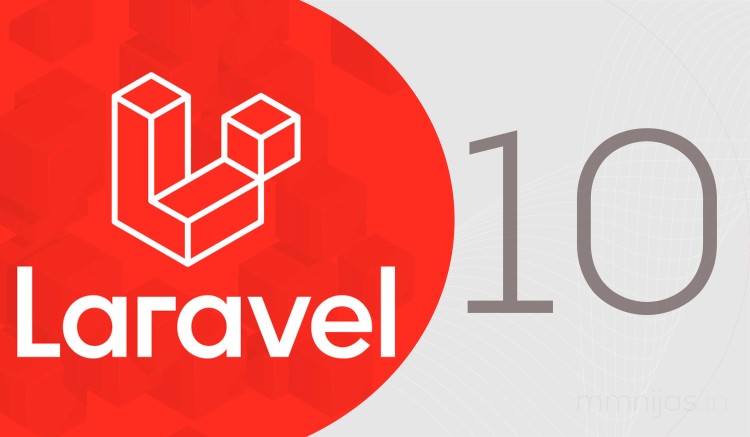
Laravel, the popular PHP framework, has recently released Laravel 10, introducing a range of exciting updates and features. In this blog post, we will explore some of the key additions in Laravel 10 with example code snippets. From performance optimizations to security enhancements and new functionalities, Laravel 10 offers developers a powerful and streamlined development experience.
Improved Performance:
a) JIT Compiler:
Laravel 10 leverages the Just-In-Time (JIT) compilation feature of PHP, resulting in improved performance. To enable JIT compilation in Laravel 10, you can use the following configuration in your php.ini file:
[opcache]
opcache.enable_cli = 1
opcache.jit_buffer_size = 100M
opcache.jit = 1205
b) Query Performance:
Laravel 10 introduces optimizations in the query builder and Eloquent ORM. For example, you can now use the latestOfMany() method to fetch the latest record from a relationship. Here's an example:
$latestComment = $post->comments()->latestOfMany()->first();
c) Blade Template Compiler Improvements:
Laravel 10 enhances the Blade template compiler for faster rendering. For instance, you can take advantage of the new @once directive to render a section of the template only once. Here's an example:
@once
<script src="https://example.com/analytics.js"></script>
@endonce
Enhanced Security Features:
a) Encrypted Cookies:
Laravel 10 introduces support for encrypted cookies to enhance security. To encrypt cookies, you can use the encryptCookies middleware in your application. Here's an example:
use Illuminate\Cookie\Middleware\EncryptCookies as Middleware;
class EncryptCookies extends Middleware
{
protected $except = [
'cookie_name',
];
}
b) Time-based CSRF Protection:
Laravel 10 includes time-based protection for CSRF prevention. This feature adds an additional layer of validation by verifying the timestamp of the request. To enable time-based CSRF protection, add the following configuration to your App\Http\Middleware\VerifyCsrfToken middleware:
protected $addHttpCookie = true;
protected $timeBased = true;
c) Security Headers:
Laravel 10 simplifies the configuration of security headers such as Content Security Policy (CSP), Strict Transport Security (HSTS), and Cross-Origin Resource Sharing (CORS). Here's an example of configuring the Content Security Policy header:
namespace App\Http\Middleware;
use Illuminate\Http\Request;
class AddContentSecurityPolicy
{
public function handle(Request $request, $next)
{
$response = $next($request);
$response->header('Content-Security-Policy', "default-src 'self'");
return $response;
}
}
New Functionalities:
a) Lazy Collections:
Laravel 10 introduces lazy collections, allowing efficient processing of large datasets. Here's an example of using a lazy collection to filter and transform data:
use Illuminate\Support\LazyCollection;
$collection = LazyCollection::make(function () {
// Fetch data from the database or any other source
return DB::table('users')->get();
})
->filter(function ($user) {
return $user->age > 18;
})
->map(function ($user) {
return $user->name;
});
foreach ($collection as $name) {
echo $name;
}
b) Async Queue Workers:
Laravel 10 introduces asynchronous queue workers for concurrent execution of queue jobs. You can dispatch jobs using the dispatchAsync() method. Here's an example:
use App\Jobs\SendEmailJob;
SendEmailJob::dispatchAsync($user, $message)->onQueue('emails');
c) Improved Routing and Route Model Binding:
Laravel 10 enhances routing and route model binding. For instance, you can now use route model binding with a custom key name. Here's an example:
Route::get('/users/{user:custom_key}', function (User $user) {
return $user;
});
Here are some bullet points on Laravel 10 - A Deep Dive Into the Latest Updates and Features:
Support for PHP 8.1: Laravel 10 requires a minimum PHP version of 8.1, and it takes advantage of a number of new features in this version of the language, such as readonly properties and array_is_list.
Route caching: Laravel 10 introduces a new feature called Route caching, which allows developers to cache their application's routes. This can improve performance by reducing the number of times that the router needs to be parsed.
Blade components: Blade components are a new way to define reusable UI elements in Laravel. They are similar to Blade views, but they are more lightweight and can be easily shared between different pages.
Packages Upgrade: Laravel 10 has upgraded a number of its official packages, including Laravel Mix, Laravel Debugbar, and Laravel Valet. These upgrades include bug fixes, new features, and performance improvements.
Predis Version Upgrade: Laravel 10 has upgraded its dependency on the Predis Redis client library to version 1.0. This upgrade includes a number of bug fixes and performance improvements.
Native Type Declarations: Laravel 10 now supports native type declarations in PHP 8.1. This means that you can now declare the types of variables and parameters in your code, which can help to improve the readability and maintainability of your code.
Laravel Pennant: Laravel Pennant is a new feature that allows developers to create and manage their own Laravel packages. Pennant provides a number of features that make it easy to create and publish packages, including a package registry, a package manager, and a package testing framework.
dispatch() Method Behavior: Laravel 10 has added a new behavior to the dispatch() method that allows developers to specify a custom middleware stack for a given job. This can be used to add additional middleware to jobs that need to be executed in a specific order or that need to be handled by a specific middleware.
Laravel 10 brings a range of updates and features to enhance performance, security, and developer productivity. By leveraging the JIT compiler, query performance optimizations, and Blade template improvements, developers can create faster and more efficient applications. The enhanced security features, including encrypted cookies and time-based CSRF protection, strengthen the security of Laravel applications. Additionally, the new functionalities such as lazy collections and async queue workers provide more flexibility and scalability. With Laravel 10, developers can build high-quality applications with ease, delivering exceptional user experiences.