Laravel Best Practices: Building High-Quality Web Applications
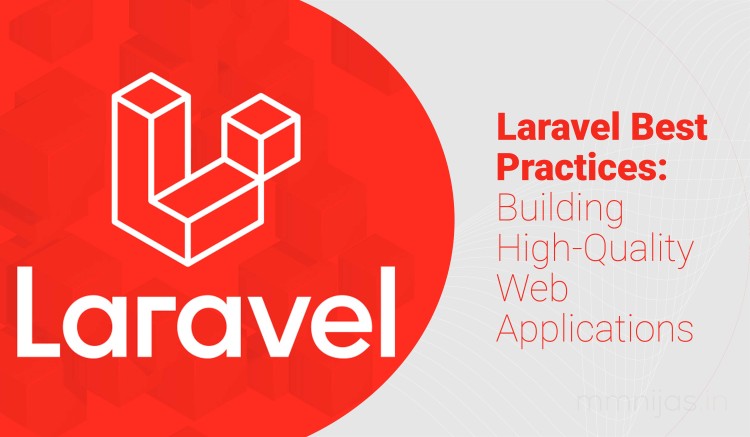
Laravel, a popular PHP framework, provides developers with a powerful and elegant toolkit for building web applications. To ensure the development of high-quality code, it is crucial to follow best practices that promote clean, maintainable, and efficient code. In this article, we will explore detailed examples of the best practices for Laravel development.
Directory Structure and Organization:
Laravel follows a well-defined directory structure that enhances organization and scalability. By adhering to this structure, developers can easily navigate and locate files. Let's take a closer look at the directories commonly used in Laravel:
app: Contains application-specific code, including models, controllers, and service classes.
resources: Stores assets like views, language files, and front-end assets.
database: Houses database-related files, such as migrations and seeders.
routes: Stores route definitions.
config: Contains configuration files for the application.
Example:
To organize your code further, you can create subdirectories within these directories based on functional modules or features. For instance, if you have an e-commerce application, you could have subdirectories like app/Http/Controllers/Shop for shop-related controllers.
Use Route Caching:
When dealing with a large number of routes, Laravel's routing system can become a performance bottleneck. Enabling route caching in the production environment helps mitigate this issue. By compiling all routes into a single file, you reduce the overhead of loading and parsing multiple files on each request, resulting in faster response times.
Example:
To cache routes, run the following command in your terminal:
Leverage Laravel Eloquent ORM:
Laravel's Eloquent ORM provides an intuitive and expressive way to interact with the database. Let's explore some best practices for using Eloquent effectively:
a) Define Relationships:
Take advantage of Eloquent's relationship system to define relationships between models. For example, if you have a User model with a one-to-many relationship with a Post model, you can define it as follows:
b) Eager Loading:
To reduce database queries and enhance performance, use eager loading when fetching related models. For instance, if you want to retrieve users along with their posts, eager load the relationship as follows:
c) Query Scopes:
Query scopes allow you to define reusable query logic within your models. They provide a convenient way to encapsulate commonly used query constraints. Here's an example of a scope to retrieve users with an active status:
Example:
To retrieve active users, you can use the defined scope as follows:
Utilize Route Model Binding:
Laravel's route model binding feature allows you to automatically inject model instances into route callbacks or controller methods. This practice simplifies your code, reduces repetitive database queries, and improves route readability.
Example:
Suppose you have a route that fetches a user based on their ID. Instead of manually fetching the user from the database, you can define the binding in your route or controller method signature. Here's an example:
Implement Caching:
Caching is a powerful technique for improving application performance. Laravel provides a robust caching system with support for various caching drivers such as Memcached and Redis. Utilize caching for frequently accessed data, such as database queries or API responses, to reduce the load on your application and improve response times.
Example:
To cache the result of a database query, you can use the remember method:
In this example, the users will be cached for the specified number of minutes. Subsequent calls to retrieve users within the cache duration will return the cached result instead of hitting the database.
Validation and Form Requests:
Validating user input is crucial to ensure data integrity and security. Laravel provides a comprehensive validation system with a wide range of validation rules. Follow these best practices:
a) Form Request Validation:
Use Laravel's Form Request feature to centralize your validation logic and keep your controllers clean. Form Requests allow you to define validation rules and authorize the request in a dedicated class.
Example:
Create a form request class using the make:request Artisan command:
In the generated class, define your validation rules and authorization logic:
In your controller method, type-hint the form request class:
b) Custom Error Messages:
Customize error messages to provide meaningful feedback to users. You can specify custom messages for each validation rule or for the entire form request.
Example:
In the form request class, add the messages method:
Error and Exception Handling:
Effective error and exception handling is essential for maintaining a stable and user-friendly application. Laravel provides a powerful exception handling mechanism. Customize and extend Laravel's exception handler to handle exceptions gracefully, log errors, and provide meaningful error responses.
Example:
To customize the exception handler, modify the report and render methods in the app/Exceptions/Handler.php file:
You can also create custom exception classes by extending the base Exception class to handle specific types of exceptions in a more specialized manner.
Implement Queues and Job Processing:
Offloading time-consuming or resource-intensive tasks to a queue and processing them asynchronously can enhance application responsiveness. Laravel's queue system, backed by drivers like Redis and Beanstalkd, allows you to dispatch jobs to a queue and process them in the background.
Example:
Create a new job using the make:job Artisan command:
In the generated job class, define the logic to be executed:
Dispatch the job using the dispatch method:
Make sure to configure your queue driver and start a worker process to process queued jobs.
Use Environment Configuration:
Laravel's configuration system supports environment-specific settings, allowing you to define different configurations for development, staging, and production environments. Utilize this feature to store sensitive information securely and keep your application flexible across different environments.
Example:
In the .env file, specify environment-specific configuration values:
Access these configuration values using the env helper function or Laravel's config helper.
Write Tests:
Testing is an essential part of any robust software development process. Laravel provides a comprehensive testing suite with various tools to facilitate testing. Let's explore some examples of testing in Laravel:
a) Unit Tests:
Unit tests focus on testing individual components of your application, such as models or helper functions. Use PHPUnit and Laravel's testing helpers to write unit tests.
Example:
b) Feature Tests:
Feature tests allow you to test the behavior of your application as a whole, simulating user interactions. Use Laravel's testing helpers and assertions to write feature tests.
Example: