Understanding Generics in Laravel: How to Simulate and Benefit from Type-Safe Flexibility
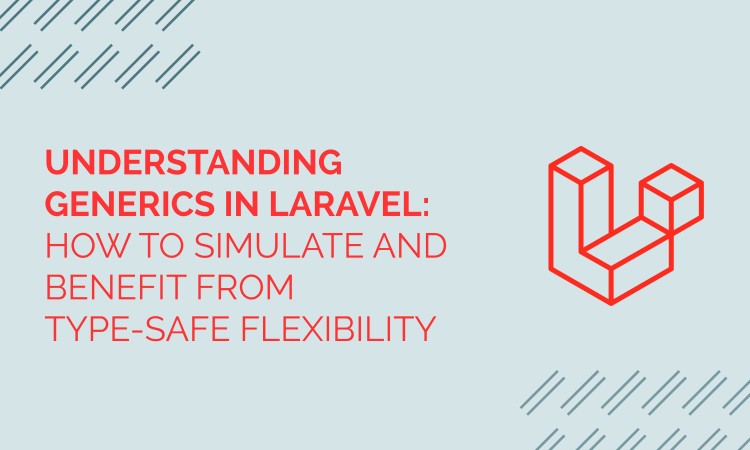
Laravel is one of the most popular PHP frameworks, known for its elegant syntax and powerful tools. However, one feature that PHP (and thus Laravel) lacks natively is generics—a concept widely used in other programming languages like Java and TypeScript to provide flexibility with strict type safety. While generics aren’t part of PHP’s core, there are ways to simulate their benefits in Laravel projects, especially when it comes to creating flexible and reusable code.
In this blog, we’ll explore what generics are, how you can achieve similar functionality in Laravel, and how tools like PHPStan or Psalm can help enforce generic-like behavior.
What Are Generics?
Generics allow developers to write code that can work with any data type while maintaining type safety. For instance, you can write a function or class that operates on an undefined type but ensure that it behaves consistently when a specific type is used.
This ensures:
- Type safety: The wrong types can’t be passed or returned.
- Reusability: The same code can work with different data types.
- Flexibility: You can extend generic code easily without rewriting it.
For example, in Java, you can create a List<T>
where T
is a placeholder for any data type. You can then create List<Integer>
, List<String>
, etc., and the compiler enforces type consistency.
Why Laravel Needs Generics
Laravel is designed to help developers write clean, maintainable code. However, as your project grows, you might find that certain patterns repeat, such as handling collections, database models, or validation. With generics, you could write flexible, reusable code that handles multiple types of data consistently, reducing boilerplate and increasing reliability.
While PHP doesn’t provide native support for generics, we can simulate generic-like functionality in Laravel using type-hinting, abstract classes, interfaces, and static analysis tools like PHPStan or Psalm.
Simulating Generics in Laravel
Even without native generics support, you can achieve a similar outcome by using some of PHP’s advanced type system features. Let’s look at how you can do this in Laravel.
1. Using Type Hinting and Abstract Classes
In Laravel, you can create abstract classes or interfaces that define the methods required for a certain type of data, while the specific implementation can vary. This allows you to write flexible code that works with various types, while enforcing consistent behavior across those types.
For instance, consider a service that operates on different types of repositories:
You can extend this base repository to work with any Laravel model:
Here, BaseRepository
serves as a generic class that can work with any Laravel model while enforcing common behavior like find()
and save()
methods.
2. Using PHPStan or Psalm for Generics-like Type Checking
Tools like PHPStan and Psalm allow you to simulate generics in PHP by adding type annotations in your code. These tools perform static analysis to check if your code conforms to the expected types, helping you catch errors early.
Consider the following example of a generic-like repository class using PHPStan annotations:
In this case, the @template T
annotation allows GenericRepository
to work with any type of model, but PHPStan will enforce that the correct types are used when calling methods like find()
and save()
.
Practical Use Case: Generic Service Layer in Laravel
Let’s consider a practical use case where a generic service layer can make your code more flexible and reusable.
Imagine you have several models like User
, Post
, and Comment
, and you want to provide CRUD operations for each of them. Instead of creating separate service classes for each model, you can create a generic service class that works for any model:
Now, you can create services for different models like this:
With this approach, you have a single CRUD service that works for any model, thanks to the generic-like flexibility provided by static analysis tools.
Benefits of Generics in Laravel
- Reusability: Write once, use everywhere. By leveraging generics, you can avoid repetitive code and reduce duplication, especially in service layers and repositories.
- Type Safety: Catch type errors early during development rather than at runtime. Tools like PHPStan and Psalm provide great support for static analysis in Laravel.
- Maintainability: Generic-like code is easier to maintain. When you need to change logic, you can update your base class or service instead of every instance.
- Scalability: As your application grows, you can introduce new models and types without needing to rewrite logic, saving time and reducing errors.
Conclusion
While PHP and Laravel don’t natively support generics, you can still simulate generic-like behavior to write flexible, reusable, and type-safe code. By leveraging Laravel's powerful type-hinting system, abstract classes, and static analysis tools like PHPStan or Psalm, you can unlock many of the benefits that generics offer in other programming languages. As PHP evolves, more advanced typing features may be introduced, but for now, these strategies provide a solid foundation for implementing generics in your Laravel applications.
Give it a try in your next Laravel project and see how much time and effort you can save by embracing this powerful concept!