Unraveling the Magic of Laravel Relationships
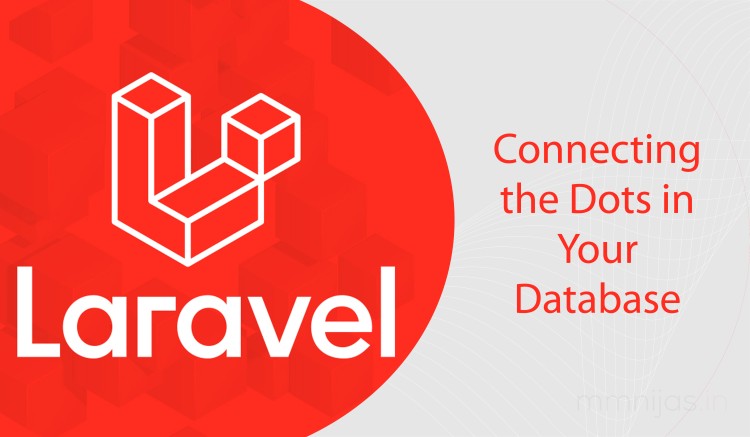
Laravel is a popular PHP framework that provides a powerful and intuitive way to build web applications. One of its key features is its support for database management and relationships between database tables. Laravel's database relationship system allows you to define and work with relationships between different database tables using object-oriented techniques.
In Laravel, relationships are defined through Eloquent, the ORM (Object-Relational Mapping) provided by the framework. Eloquent simplifies database operations by allowing you to work with database records as objects and define relationships between them using familiar object-oriented principles.
There are several types of relationships you can define in Laravel:
One-to-One Relationship: This relationship is used when a model has exactly one related model. For example, a User model may have one associated Profile model.
One-to-Many Relationship: In this type of relationship, a model can have multiple related models. For example, a User model may have multiple associated Post models.
Many-to-One (N:1) Relationship: This is the inverse relationship of a one-to-many relationship. Many records on the "many" side are associated with a single record on the "one" side.
Many-to-Many Relationship: This relationship is used when multiple models are related to multiple other models. For example, a User model may be associated with multiple Role models, and a Role model may be associated with multiple User models.
Has Many Through Relationship: This relationship allows you to define a relationship that involves traversing through another model. For example, you can define a relationship between a Country model and a User model, where the User model belongs to a City model, which in turn belongs to a Country model.
Polymorphic Relationships: Polymorphic relationships are used when a model can belong to more than one other model on a single association. For example, a Comment model can belong to either a Post model or a Video model.
These are the main types of relationships supported by Laravel's Eloquent ORM. Each relationship type has its own methods and conventions for defining and working with related data. It's important to understand these relationship types and how they can be used to model the data structure of your application efficiently.
One-to-One (1:1)
Certainly! A One-to-One relationship is a type of relationship in database design where each record on one side of the relationship is associated with at most one record on the other side. In Laravel's Eloquent ORM, a One-to-One relationship allows you to define a relationship between two database tables where one record on the "one" side is associated with at most one record on the "other" side.
Let's consider an example to understand the One-to-One relationship. Suppose we have two tables: "users" and "profiles". Each user has one profile, and each profile belongs to only one user. In this scenario, we have a One-to-One relationship between the "users" and "profiles" tables.
To define this relationship in Laravel, we'll use the Eloquent models for the "users" and "profiles" tables. Let's assume we have a User model and a Profile model.
In the User model, we'll define a method called profile to represent the relationship. Within this method, we'll use the hasOne method provided by Eloquent:
In the Profile model, we'll define a method called user to represent the inverse side of the relationship. This is done using the belongsTo method:
With these relationship definitions, we can now easily work with the One-to-One relationship between users and profiles.
For example, we can retrieve the profile associated with a user by calling the profile method on a User instance:
Similarly, we can access the user associated with a profile by calling the user method on a Profile instance:
These methods allow us to navigate the relationship and retrieve the related records effortlessly.
One-to-Many (1:N)
One-to-Many (1:N) relationship is one of the fundamental types of relationships in database design. In the context of Laravel's Eloquent ORM, a One-to-Many relationship allows you to define a relationship between two database tables where one record on the "one" side is associated with multiple records on the "many" side.
Here's an example to help illustrate the concept:
Let's say we have two tables in our database: "users" and "posts". Each user can have multiple posts, but each post belongs to only one user. In this scenario, we have a One-to-Many relationship between the "users" and "posts" tables.
To define this relationship in Laravel, we'll use the Eloquent models for the "users" and "posts" tables. Let's assume we have a User model and a Post model.
In the User model, we'll define a method called posts to represent the relationship. Within this method, we'll use the hasMany method provided by Eloquent:
In the Post model, we'll define a method called user to represent the inverse side of the relationship. This is done using the belongsTo method:
With these relationship definitions, we can now easily work with the One-to-Many relationship between users and posts.
For example, we can retrieve all the posts associated with a user by calling the posts method on a User instance:
Similarly, we can access the user associated with a post by calling the user method on a Post instance:
These methods allow us to navigate the relationship and retrieve the related records effortlessly.
Many-to-One (N:1)
A Many-to-One (N:1) relationship, also known as a "belongsTo" relationship, is the inverse of a One-to-Many relationship. In this type of relationship, multiple records on the "many" side are associated with a single record on the "one" side.
Let's continue with the previous example of the "users" and "posts" tables to illustrate a Many-to-One relationship. In this scenario, each post belongs to a single user, but a user can have multiple posts. This relationship is Many-to-One because many posts belong to one user.
To define a Many-to-One relationship in Laravel's Eloquent ORM, we need to set up the appropriate methods in the Post and User models.
In the Post model, we'll define a method called user to represent the relationship with the "users" table. We'll use the belongsTo method to define the relationship:
In the User model, we don't need to define any methods as we did in the One-to-Many relationship. The framework assumes that the foreign key for the Many-to-One relationship is stored on the "many" side, which is the posts table in this case. By default, Laravel assumes that the foreign key column is named user_id (the singular form of the related model, followed by _id).
Now, we can easily retrieve the user associated with a post:
In this example, calling $post->user will return the User model associated with that post.
With Many-to-One relationships, you can perform various operations, such as querying for posts belonging to a specific user or accessing attributes of the associated user. The relationship allows you to navigate between the related tables efficiently and retrieve the necessary data.
Many-to-Many (N:N)
A Many-to-Many (N:N) relationship is a type of relationship where multiple records on one side of the relationship can be associated with multiple records on the other side. In Laravel's Eloquent ORM, this relationship is implemented using a pivot table to store the associations between the two related tables.
Let's consider an example to understand the Many-to-Many relationship. Suppose we have two tables: "users" and "roles". Multiple users can have multiple roles, and each role can be assigned to multiple users. This scenario represents a Many-to-Many relationship between the "users" and "roles" tables.
To define a Many-to-Many relationship in Laravel, we'll need three tables: the two related tables ("users" and "roles") and a pivot table that will store the associations.
In Laravel's Eloquent ORM, we'll have three models: User, Role, and a pivot model, let's call it UserRole.
Here's an example of how we can define the Many-to-Many relationship using these models:
In this example, we define a belongsToMany relationship in both the User and Role models. The belongsToMany method takes two parameters: the related model (e.g., Role::class) and the name of the pivot table (user_roles).
Now, we can easily work with the Many-to-Many relationship. For example, we can retrieve all the roles associated with a user:
Conversely, we can access all the users assigned to a role:
We can also perform additional operations, such as attaching and detaching records in the Many-to-Many relationship using the attach, detach, and sync methods provided by Laravel.
Has Many Through
The "Has Many Through" relationship in Laravel allows you to define a relationship that involves traversing through another model. It provides a way to define a relationship between two models indirectly through a third model.
Let's consider an example to understand the "Has Many Through" relationship. Suppose we have three tables: "countries", "cities", and "users". Each country has many cities, and each city has many users. However, we want to define a relationship between the "countries" and "users" tables directly, without having to define a direct relationship between them.
To achieve this, we can use the "Has Many Through" relationship in Laravel. We'll need three models: Country, City, and User.
Here's an example of how we can define the "Has Many Through" relationship using these models:
In this example, we define the "Has Many Through" relationship in the Country model using the hasManyThrough method. The hasManyThrough method takes two parameters: the related model (User::class) and the intermediate model (City::class).
With this relationship defined, we can now easily retrieve all the users associated with a country:
The hasManyThrough relationship works by automatically performing a join operation on the intermediate cities table to retrieve the related users. It saves us from having to explicitly define a direct relationship between the Country and User models.
Polymorphic Relationship
A Polymorphic relationship in Laravel's Eloquent ORM allows a model to belong to multiple other models on a single association. It provides a way to create flexible and generic relationships where a model can be associated with various other models.
In a Polymorphic relationship, there are three main components:
Morphable Model: This is the model that can belong to multiple other models. It defines the polymorphic relationship and contains the morphable columns.
Morphable Columns: These are the columns in the morphable model's database table that store the related model's information. The morphable columns typically consist of two parts: the morphTo column, which stores the related model's type, and the morphId column, which stores the related model's primary key.
Related Models: These are the models that can be associated with the morphable model. Each related model needs to define a morphMany or morphOne relationship to establish the polymorphic relationship.
Let's illustrate the Polymorphic relationship with an example. Suppose we have three models: Image, Video, and Comment. Each model can have multiple tags associated with it. Instead of creating separate tables for tags for each model, we can use a Polymorphic relationship to achieve this flexibility.
Here's an example of how we can define the Polymorphic relationship using these models:
In this example, the Tag model defines the polymorphic relationship using the morphTo method. The Image, Video, and Comment models define a morphMany relationship to establish the association with the tags.
Now, we can easily work with the Polymorphic relationship. For example, we can retrieve all the tags associated with an image:
Similarly, we can access the tags associated with a video or a comment in the same way.
The Polymorphic relationship provides a flexible way to associate a model with multiple other models using a single association. It allows you to easily extend the relationships and avoid creating separate tables for each related model.